In this Reactive Forms tutorial, you will learn everything about how to create a dynamic radio list component in an Angular application. Using this Radio List form field component, you can easily create multiple radio groups on a single page.
The best part of our Radio list component is that it will use the Reactive Forms API to create robust and scalable forms which can have other dynamic components as well like Select Dropdown Components.
Moreover, it will support various other required functionalities like required validation message when no values is selected on submit, passing a dynamic list of radio items and selecting default value.
Our Radio List Reactive component will support various Input params to make it more flexible to be used which are listed below:
- A dynamic list of radio list items passes from the parent component.
- Pass the default selection value (Optional)Â of the radio item in the list
- Required validation(Optional) on radio list using param.
We will create a Reusable Radio List Component that will use the Reactive Forms approach, then this component will be using the parent component to be a part of FormGroup for creating scalable forms.
Let’s start building the Radio List Component…
How to Create a Radio List Component using Reactive Forms in Angular 15?
Follow these easy steps to setup an Angular app then create the Radio list component:
Step 1 – Create an Angular App
Step 2 – Update App Module
Step 3 – Include Bootstrap Library
Step 4 – Create a Radio List Component
Step 5 – Using Radio List Component in Parent
Step 6 – Run the Application
Step 1 – Create an Angular App
Before creating the Angular app, you can install the latest version of the Angular CLI tool to have the latest version by executing the following command in the terminal:
npm install -g @angular/cli
Now you are ready to create a new Angular app by executing the ng new command with the application name provided below:
ng new radio-list-app
Enter inside the application directory
cd radio-list-app
Step 2 – Update App Module
To use the Reactive Forms in our Angular project, you need to import the ReactiveFormsModule which are part of Angular Forms.
hereafter, add it to the imports array as shown below:
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { ReactiveFormsModule } from '@angular/forms';
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule, AppRoutingModule, ReactiveFormsModule],
providers: [],
bootstrap: [AppComponent],
})
export class AppModule {}
Step 3 – Include Bootstrap Library
In our app, we will use Bootstrap CSS to style the components quickly. Install the Bootstrap by executing below command:
npm install bootstrap
After installation is complete, open the angular.json file and update the styles
array to add a bootstrap css file as shown below:
...
"styles": [
"node_modules/bootstrap/dist/css/bootstrap.min.css",
"src/styles.css"
],
...
Step 4 – Create a Radio List Component
Now, we will create the Radio list component by executing the below ng command:
ng generate component reusable-radio-list
Thereafter, update the component class and HTML template as shown below:
reusable-radio-list.component.ts
import { Component, Input, OnInit } from '@angular/core';
import { FormControl, FormGroup } from '@angular/forms';
@Component({
selector: 'app-reusable-radio-list',
templateUrl: './reusable-radio-list.component.html',
styleUrls: ['./reusable-radio-list.component.css'],
})
export class ReusableRadioListComponent implements OnInit {
@Input() options: any[] = [];
@Input() controlName!: string;
@Input() parentForm!: FormGroup;
@Input() control!: FormControl;
@Input() enableValidation = true;
constructor() {}
ngOnInit(): void {}
}
reusable-radio-list.component.html
<div [formGroup]="parentForm">
<div class="form-check" *ngFor="let option of options">
<input class="form-check-input" type="radio" [value]="option.value" [formControlName]="controlName"
[id]="controlName + '-' + option.value" />
<label class="form-check-label" [for]="controlName + '-' + option.value">
{{ option.label }}
</label>
</div>
<div *ngIf="control?.touched && control?.invalid" class="text-danger">
Please select an option.
</div>
</div>
In the class component, we have defined various @Input param, which will be passed by the parent component to configure our Radio Lists. Following are the details about various input properties used:
Input Parameter | Description |
---|---|
options | An array of options to be displayed in the radio button list. |
controlName | The name of FormControl is associated with the radio button list in the parent FormGroup. |
parentForm | The parent FormGroup contains the FormControl for the radio button list. |
control | The FormControl associated with the radio button list, is used for accessing validation states. |
enableValidation | A boolean flag to determine if the required validation should be enabled for the radio list. |
In the HTML template, we used Bootstrap classes to style the Radio list items and also there is error text to be shown when the Radio list is required and left empty after clicking the Submit button in the parent component.
Step 5 – Using Radio List Component in Parent
We are now ready to consume our Radio list component in the parent component. We will use it inside the App Component.
Open the app.component.html file and update below:
<div class="container mt-5">
<div class="row">
<div class="col-md-6">
<div class="card">
<div class="card-body">
<h2 class="mb-4">Radio Group lists using Reactive Forms in Angular</h2>
<form [formGroup]="exampleForm" (ngSubmit)="onSubmit()">
<h5>Choose a favorite color:</h5>
<app-reusable-radio-list
[options]="colorOptions"
controlName="favoriteColor"
[parentForm]="exampleForm"
[control]="favoriteColorControl"
[enableValidation]="enableColorValidation"
></app-reusable-radio-list>
<h5>Choose a favorite fruit:</h5>
<app-reusable-radio-list
[options]="fruitOptions"
controlName="favoriteFruit"
[parentForm]="exampleForm"
[control]="favoriteFruitControl"
></app-reusable-radio-list>
<button type="submit" class="btn btn-primary mt-3">Submit</button>
</form>
</div>
</div>
</div>
</div>
</div>
Now update the app.component.ts file:
import { Component } from '@angular/core';
import {
FormBuilder,
FormControl,
FormGroup,
Validators,
} from '@angular/forms';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
exampleForm: FormGroup;
enableColorValidation = false; //Disable Required validation on Colors Radio List
colorOptions: any[] = [
{ label: 'Red', value: 'red' },
{ label: 'Blue', value: 'blue' },
{ label: 'Green', value: 'green' },
];
fruitOptions: any[] = [
{ label: 'Apple', value: 'apple' },
{ label: 'Banana', value: 'banana' },
{ label: 'Cherry', value: 'cherry' },
];
constructor(private fb: FormBuilder) {
this.exampleForm = this.fb.group({
favoriteColor: [
'blue',
this.enableColorValidation ? Validators.required : null,
],
favoriteFruit: ['', Validators.required],
});
}
get favoriteColorControl(): FormControl {
return this.exampleForm.get('favoriteColor') as FormControl;
}
get favoriteFruitControl(): FormControl {
return this.exampleForm.get('favoriteFruit') as FormControl;
}
onSubmit(): void {
if (this.exampleForm.valid) {
const selectedColor = this.exampleForm.get('favoriteColor')?.value;
const selectedFruit = this.exampleForm.get('favoriteFruit')?.value;
alert(
`Selected Color: ${selectedColor}\nSelected Fruit: ${selectedFruit}`
);
} else {
this.exampleForm.markAllAsTouched();
console.log('Form is not valid.');
}
}
}
We have defined colorOptions
and fruitOptions
to create two Radio Lists which will be passed as param in the <app-reusable-radio-list>
component.
Inside the constructor, we have defined form builder group and decided which radio list needs to be validated for the required selection.
There are getters for each radio list to control error message visibility.
The onSubmit()
function will validate the form and show the values in the alert box.
Step 6 – Run the Application
We have completed the Radio List building process. Now you can execute the npm start
command to start the webserver and run the application.
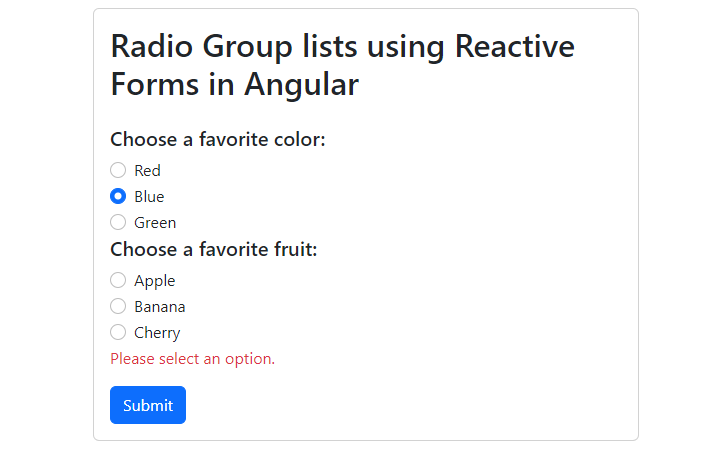
Conclusion
We have successfully created a reusable radio button list component using Angular Reactive Forms. This versatile component can dynamically generate multiple radio groups with customizable options, allowing for easy integration into various forms.
By utilizing the input parameters, we have achieved a high level of customization, sch as setting default values, enabling or disabling required validation, and configuring the options for each radio group.
Additionally, the component seamlessly integrates with parent forms, making it convenient to access and manage form controls and validation states. This component can be used to kickstart and enhance the feature as per requirements. hope this will be helpful …
Leave a Reply